Java, like many object-oriented programming languages, embraces the concept of abstraction. Abstraction allows programmers to focus on the "what" rather than the "how" of an object's behaviour. Abstract methods are a powerful tool that embodies this principle. In this article, we will talk about abstract methods in Java, their examples, when to use them, and more!
What are Abstract Methods in Java?
An abstract method in Java is a method declared with the abstract keyword that lacks an implementation (body). It essentially outlines the functionality a subclass or implementing class must provide. Abstract methods are typically found within abstract classes or interfaces. They allow a class to define a protocol for its subclasses to follow without providing a complete implementation. This allows for code reuse and facilitates polymorphism.
Key Characteristics of Abstract Methods
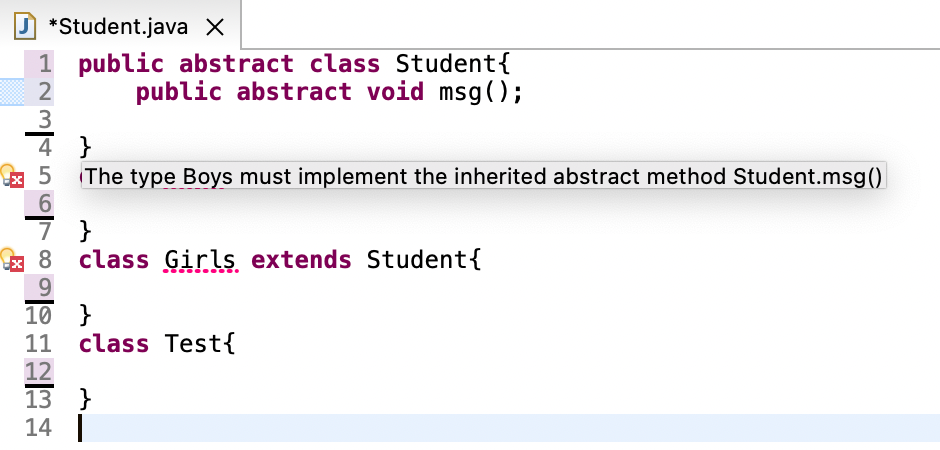
- Declared with “abstract” keyword: This keyword signifies the method doesn't have a concrete implementation in the current class.
- No method body: The curly braces “{}” are absent, indicating the specific steps to execute the functionality are missing.
- Defines method signature: The method signature includes the return type, name, and parameter list, specifying the expected input and output.
Implemented by subclasses/implementing classes: Subclasses inheriting from an abstract class or classes implementing an interface must provide a concrete implementation for all abstract methods.
Read More: 100+ Java interview questions for experienced developers
Examples of Abstract Methods in Java
Let's design a program to calculate areas of various shapes. We can create an abstract class named "Shape" with an abstract method "calculateArea()":
This "Shape" class defines the concept of a shape but doesn't specify how to calculate the area. Subclasses representing specific shapes like "Circle," "Rectangle," and "Triangle" will inherit from "Shape" and implement "calculateArea()" with their unique formulas.
Here's how it plays out:
- Abstract Class (Shape): The "Shape" class acts as a blueprint for specific shapes. It outlines the "calculateArea()" method but doesn't provide the steps to calculate it. This method signature tells subclasses what the method should do (return a double representing the area) but leaves the "how" for them to implement.
- Subclasses (Circle, Rectangle, Triangle): These subclasses inherit from "Shape" and are responsible for providing concrete implementations for "calculateArea()".some text
- Circle: The "Circle" class will likely use the formula "PI * radius * radius" to calculate its area.
- Rectangle: The "Rectangle" class might use "length * breadth" to determine its area.
- Triangle: The "Triangle" class might implement a formula based on its sides or angles to calculate its area.
So, with the help of an abstract method, a common functionality for all shapes is defined. And, the subclasses can handle the specifics based on their geometry.
Abstract Methods in Java Interface
Interfaces play an important role in Java by defining contracts for object behavior. Unlike abstract classes, interfaces exclusively feature abstract methods. These methods serve as placeholders.
Here's a breakdown of how abstract methods work within interfaces:
- Implicitly Abstract: Any method declared within an interface is inherently abstract. You don't explicitly use the abstract keyword when defining these methods.
- No Implementation: Interfaces focus on "what" needs to be done, not "how." Therefore, abstract methods within interfaces lack an implementation body (curly braces {}). They only specify the method name, return type, and parameter list.
- Implemented by Concrete Classes: Classes that "implement" an interface are responsible for providing concrete implementations for all the abstract methods declared within the interface. This ensures that any object referencing the interface can call these methods and expect the functionality defined by the concrete class.
In essence, interfaces with abstract methods establish a blueprint for how objects should behave. Concrete classes adhering to the interface contract fill in the details, creating a more flexible and modular design.
Important Points for Java Abstract Methods
Here are some key aspects to remember when working with abstract methods in Java:
- Abstract classes cannot be instantiated: You cannot directly create objects of an abstract class. They serve as base classes for inheritance.
- Subclasses must override abstract methods: A subclass inheriting from an abstract class must provide implementations for all inherited abstract methods. If not, the subclass itself needs to be declared abstract.
- Final methods cannot be abstract: A method declared as final cannot be overridden, and hence, it cannot be abstract as well.
- Abstract methods can have access modifiers: You can use access modifiers like public, protected, or private with abstract methods to control their visibility.
Benefits of using Abstract Methods in Java
Abstract methods are useful in designing frameworks and APIs where the behavior of specific methods can vary based on the implementation.
- Promote Code Reusability: By defining a common interface through abstract methods, you encourage code reuse. Subclasses can leverage the existing functionality defined in the abstract class or interface, reducing code duplication.
- Enforce Code Contracts: Interfaces with abstract methods establish contracts that concrete classes must adhere to. This ensures a level of consistency and predictability in how objects behave.
- Facilitate Polymorphism: Abstract methods enable polymorphism, a core principle of object-oriented programming. Polymorphic behaviour allows treating objects of different subclasses in a similar way as long as they implement the same abstract method. This makes code more flexible and adaptable.
- Promote Loose Coupling: This means the abstract methods ensure that subclasses remain independent. The primary focus lies in fulfilling the functionality outlined by the abstract methods.
When to use Abstract Methods?
- Defining Common Functionality for Related Classes: When a group of classes share some core functionality but have variations in specific implementations, an abstract class with abstract methods can be a good choice. Subclasses can then inherit the common functionality and provide their unique implementations for the abstract methods.
- Creating Templates for Object Behavior: Abstract methods can act as templates, outlining the expected behaviour for a specific task. Subclasses then fill in the details based on their specific needs.
- Enforcing Contracts with Interfaces: Interfaces with abstract methods are ideal for defining contracts that concrete classes must follow. This ensures consistency and promotes code maintainability.
Ace Your Java Interviews with Interview Kickstart Today!
Want to land your dream job at a top tech company? Interview Kickstart can help you get there. We offer a powerful combination of expert guidance and real-world practice.
Learn from over 500 instructors with experience at FAANG companies, master essential Java skills with our comprehensive curriculum, and gain confidence through live training sessions and mock interviews.
Over 17,000 tech professionals have transformed their careers with Interview Kickstart. Ready to join them? Register for our free webinar today and learn how we can help you to nail your Java interview.
Key Takeaway -
Abstract methods in Java define functionality without implementation, promoting code reusability, polymorphism, and loose coupling. They help you create well-structured and adaptable object-oriented programs.
Abstract Methods in Java FAQs
- What's the difference between an abstract method and a regular method?
Regular methods have a defined implementation (body) with specific instructions. Abstract methods lack an implementation and only specify the method signature (return type, name, and parameters).
- Can an Interface have concrete methods?
No, interfaces cannot have concrete methods. All methods declared within an interface are implicitly abstract, meaning they lack an implementation.
- Why can't you create objects of an abstract class?
Abstract classes serve as blueprints for inheritance. They often lack complete functionality due to abstract methods. Since these methods require implementation, you cannot directly create objects of an abstract class.
- Is it mandatory to override all inherited abstract methods in a subclass?
Yes. If a subclass inherits from an abstract class, it must provide implementations for all inherited abstract methods. Otherwise, the subclass itself needs to be declared abstract.
- Do I have to implement all methods in an abstract class if I inherit from it?
Yes, if you subclass an abstract class, you must provide implementations for all inherited abstract methods. Otherwise, your subclass itself needs to be declared abstract.